A Lightweight Image Comparison Library
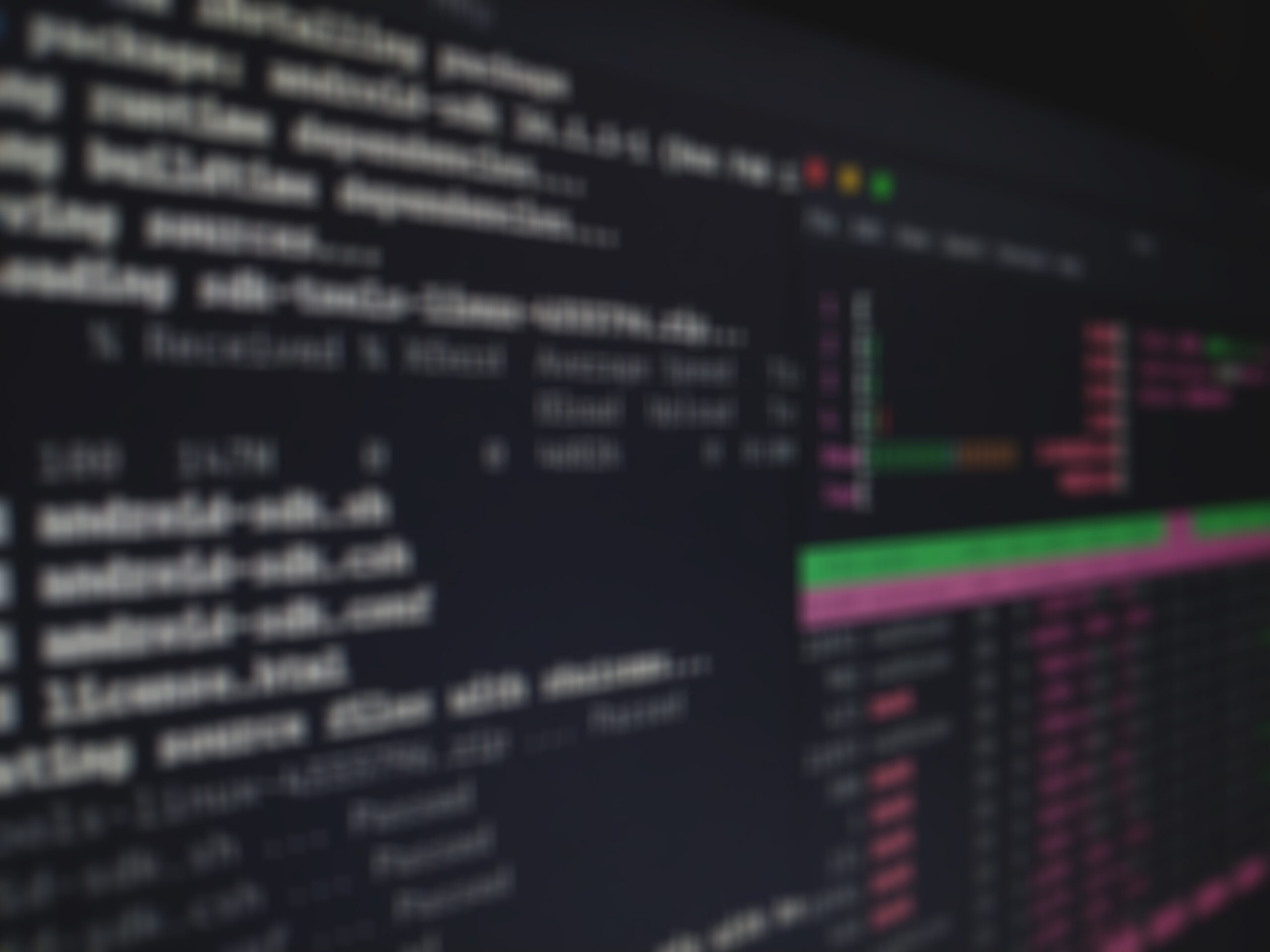
QGoImageCompare was developed in the Megason Lab at Harvard Medical School and at the University of Wisconsin-Madison. It is a library aimed at simple comparison of images. The library provides an easy means to add 2D and 3D image visualization and examination features to a Qt4 application, or the bundled visualization executables can be used on their own.
This project merged with Matt McCormick’s visual debugger for ITK in order to provide a complete package for visualizing images while debugging an ITK pipeline. The developers are now from the many itk::ImageFileWriter’s in their code.
QGoImageCompare can be used in the process of developing an ITK or VTK image processing or data visualization program and developers can use it to quickly visualize and compare results of filters in different parts of the pipeline. It is capable of displaying images directly from the memory while debugging an ITK application. Finally, QGoImageCompare can be used in software applications like Slicer, SNAP, GoFigure2 or V3D in their plug-ins infrastructure [1] to compare the input and the resulting output of one filter.
QGoImageCompare is hosted on a github repository [2]. The repository includes code source, documentation, publication, examples (in /Examples/GUI/lib/), tests (in /Testing/GUI/), and executables / applications (in /Main/).
Features
QGoImageCompare includes:
• Support for ITK scalar images (see supported format for ITK image support details)
• Support for VTK images
• 2D or 3D images with planar views and a tri-planar (xy, xz, yz) 3D view
• Camera synchronization for fine comparison of results
• Choice of look-up tables
• Pixel examination, location, index, and value at cross-hair
• QWidget UI form for easy customization using Qt Designer
• QWidget inheritance for integration to a Qt GUI based application
• A manager class that takes care of visualizations properties
(creation, deletion, synchronization of several views, look-up tables…)
• ITK visual comparison debugging
Visualization Library
The library is composed by six classes, which are all Qt objects.
The abstract class for QGoSynchronizedView2D and 3D is QGoSynchronizedView. It is used to display a QWidget containing a two- or three- dimensional vtkImageData* or itk::Image*. It provides the interface to synchronize cameras among several of the abstract classes’ objects.
QGoSynchronizedView2D(3D)Callbacks takes a list of the abstract 2D and 3D classes and synchronizes their cameras by setting up callbacks. It is highly recommended to let the QGoSynchronizedViewManager handle this process.
QGoSynchronizedViewManager is the high level class for the QGo 2D and 3D synchronized views and callbacks. This class deals with QGoSynchronizedViews for correct synchronization and provides a simple interface to create, delete and synchronize QGoSynchronizedViews.
For more details, we invite you to look at the Doxygen documentation and the source code.
Library Usage
For further clarification, there is a description of the basic use of the visualization library and an example to illustrate its functionality.
Code Snippets
We introduce here the high-level functions for creating QWidgets views and synchronizing visualizations, based on the QGoSynchronizedViewManager.
To develop a visualization manager object we simply create a new manager that will take care of creation/deletion of visualization and callbacks for us.
QGoSynchronizedViewManager* ViewManager = new QGoSynchronizedViewManager(); |
We can now visualize and process images as necessary. Once visualization is no longer needed, remember to delete ViewManager.
delete ViewManager; |
For the visualization of a VTK image, the synchronization manager can create visualization windows given a valid pointer to the image and a string encoding the name of the visualization.
ViewManager->newSynchronizedView(“My VTK View”, VTKSmartPointerToImage); ViewManager->Update(); ViewManager->show(); |
For the visualization of an ITK image, the synchronization manager can create visualization windows given a valid pointer to the image, the template argument representing the image pixel type and a string encoding the name of the visualization.
ViewManager->newSynchronizedView<InputPixelType>( “My ITK View”, ITKSmartPointerToImage); ViewManager->Update(); ViewManager->show(); |
The camera for several open images can be synchronized by the synchronization manager with a simple function call.
ViewManager->synchronizeOpenSynchronizedViews(); |
Examples
Here, we illustrate a simple segmentation pipeline design (Figure 1), for ear cell nuclei in developing zebrafish embryo (Figure 2(a)). We show synchronized images at each step of the pipeline (Figures 2(a), 2(b), 2(c)).
Note that a possible improvement could be to add one QWidget for each filter with sliders or spin boxes to tweak each parameter involved in this process.
Figure 1: The diagram shows how to use the code snippets to visualize intermediary results in an
image-processing pipeline. (The example can be found in /Examples/GUI/lib/pipeitkexample.cxx)
Figure 2: Viewers generated by pipeitkexample, showing (a)the input image,
(b) the filtered image, and (c) the thresholded image.
The pipeitkexample takes a 3D image as an argument, and processes it, displaying the output of the filters at each stage of the pipeline in a synchronized manner.
The pipevtkexample takes vtk readable image as an argument and blurs it. It displays the input and the output of the filter in a synchronized manner.
Applications
comparegui shows how to create a very basic GUI using the functionalities provided by the QGoSynchronized classes. Figure 3 is a screenshot of this application. comparesimple takes a list of 2D or 3D images as an input and displays them in synchronized viewer widgets.
Figure 3: Illustration of comparegui example application. We use it to compare two
identical datasets, in a synchronized manner, with a custom look-up table.
Visual Debugging
Here we describe the GDB-pretty-ITK project, which utilizes QGoImageCompare for debugging image-processing pipelines using the GNU Debugger (GDB). When creating a new ITK pipeline or filter, it is often beneficial to examine the intermediate images that are generated. In the context of a debugging, pretty-printing is the act of displaying a complex data structure in an insightful way. For example, pretty-printing a C array pointer may involve printing the elements of the array or pretty-printing a C structure may imply printing the structure’s members. In a previous article in the Source [3], we demonstrated how custom GDB Python pretty-printers could be used to launch an image viewer, which displays an itk::Image’s BufferedRegion when ‘printing’ the object in GDB.
In our previous work, we launched an independent child process such as ParaView or Mayavi for each object that was printed. Here we describe a new Python pretty-printer based on QGoImageCompare. This pretty-printer utilizes the well-designed visualization and analysis tools of QGoImageCompare. It also has an advantage over the other image viewer pretty-printers because the camera view is synchronized between all images that are printed.
The first time print is called on an itk::Image pointer, the pretty-printer module, called icp, launches a child process, icpGui. A separate process is needed to separate the GDB event loop and the Qt event loop. The image’s information (spacing, origin, etc.) and data buffer are passed from the Python module to the GUI via Qt’s inter-process communication (IPC) capabilities. Subsequent images are sent across the same IPC channels and the view is synchronized between images. Simultaneous synchronized zooming, for instance, can be very helpful in image analysis.
The GDB-pretty-ITK project is still in the alpha stages, but it is usable and may be helpful in your adventures with ITK. We have tried to lower the barrier to installation and usage, although there is admittedly a way to go. A set of installation instructions has been placed on the ITK wiki [4]. Instructions exist for the QGoImageCompare pretty-printer along with the rest of the system. Note that the entire system must be installed to use the icp pretty-printer. We also developed a set of CMake modules to ease registration of the ITK pretty-printers in your project [5].
An example use of the icp pretty-printer is distributed as a CTest test within the source tree. This example includes a simple executable and a GDB script of commands that can be interactively entered into the debugger.
References
[1] Chen, S. Insight Toolkit Plug-ins: Volview and V3D, Kitware Source, October 2010.
[2] QGoImageCompare: Source code of QGoImageCompare. https://github.com/gofigure2/QGoImageCompare.
[3] McCormick, M. Visual Debugging of ITK. Kitware Source. April 2010.
http://kitware.com/products/html/VisualDebuggingOfITK.html
[4] ITK/GDBPretty. The ITK Wiki. http://www.itk.org/Wiki/ITK/GDBPretty.
[5] gdb-pretty-itk-cmake: CMake scripts to register GDB pretty-printers for ITK on targets.
http://gitorious.org/gdb-pretty/gdb-pretty-itk-cmake.
Acknowledgements
This work was supported by NHGRI P50 HG004071 and NIDCD R01 DC010791.
Antonin Perrot-Audet is a Ph.D. student at the CREATIS Lab, University of Lyon in France, and in collaboration with the Megason Lab at Harvard Medical School, working on his thesis in registration of cell lineages.
Matthew McCormick is a PhD. candidate at the University of Wisconsin-Madison working on his thesis in medical ultrasound characterization of carotid plaques.
Arnaud Gelas is a Research Associate in the Megason Lab at Harvard Medical School where he is in charge of the GoFigure2 project.
Nicolas Rannou is a Research Associate in the Megason Lab at Harvard Medical School where he is participating in the development of GoFigure2. He is currently in charge of the Visualization component in GoFigure2.
Lydie Souhait is a Research Associate in the Megason Lab at Harvard Medical School where she is participating in the development of GoFigure2. She is currently in charge of the Database and GUI components in GoFigure2.
Kishore Mosaliganti is a Research Fellow in the Megason Lab at Harvard Medical School where he is currently developing algorithms for the extraction of zebrafish ear lineages using confocal microscopy images to be included in GoFigure2.
Sean Megason is an Assistant Professor in the Department of Systems Biology at Harvard Medical School. The Megason lab wants to understand how the code in the genome is executed to turn an egg into an embryo.