Convert ITK Data Structures to NumPy Arrays
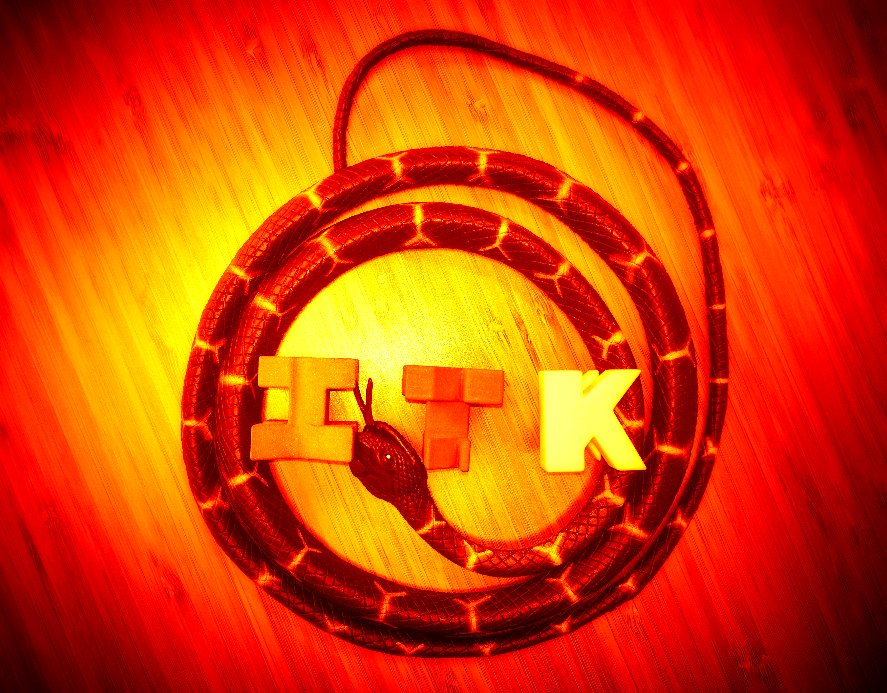
We previously announced that the ITK Python wrapping is now available in the Python Package Index (PyPI) [1].
Starting to develop Python scripts using ITK filters is one command line away:
python -m pip install itk
Python is a scripting language that allows fast development and is widely used in the scientific community.
You can use ITK Python to read and write all the common image formats as well as many others. Combined with the power and speed of the hundreds of ITK filters, it is fast and easy to create and adapt complex image processing pipelines. The example below illustrates how to create a simple pipeline that reads an image, processes it with a median filter, and saves the result.
import itk image = itk.imread("input_filename.png") median = itk.MedianImageFilter.New(image, Radius = 2) itk.imwrite(median, "output_filename.png")
Sometimes, such as when one wants to display an image in an IPython notebook, it is necessary to directly access the raw content of an image. It is possible through the ITK image object to get the image values pixel by pixel, but manually iterating over the entire image would result in slow processing. Instead, ITK provides a bridge to convert ITK data to NumPy arrays. The conversion can be performed in both directions. It is therefore possible to combine the speed and power of ITK to process images, and the speed and versatility of NumPy to accomplish specific operations that are not available in ITK.
The ITK NumPy bridge converts ITK images, but also vnl vectors and vnl matrices to NumPy arrays. The data can either be copied into a new object or a view on the data can be created. The view allows access and modification of the data without the need to duplicate its memory. Depending on the situation, a view or copy is preferred.
The functions to convert ITK images to/from NumPy arrays/views are:
image_view = itk.GetImageViewFromArray( np_array) image = itk.GetImageFromArray( np_array) array_view = itk.GetArrayViewFromImage(image) array = itk.GetArrayFromImage(image)
All the functions to convert to and from vnl vectors and vnl matrices are presented in the ITK Python Quick Start Guide [2] (Hint: It is easy to guess their names).
A short example illustrating how to use ITK Python and the ITK NumPy bridge is available here [3].
Have fun using ITK!
[1] https://blog.kitware.com/itk-is-on-pypi-pip-install-itk-is-here
[2] https://itkpythonpackage.readthedocs.io/en/latest/Quick_start_guide.html
[3] https://github.com/fbudin69500/ConvertITKDatatoNumPyArrayBlogPost
What’s the difference between this and SimpleITK? What’s recommended?
Hello Fernando. SimpleITK is a simplified layer built on top of ITK. It is wrapped in many languages such as Python and R and has been available for a while. It is also available on CondaForge which makes it easy for people using that platform to download it and install it.
ITK Python that is presented in this blogpost is the direct Python wrapping of ITK and gives access to all the algorithms in ITK. Everything that is in ITK is available in ITK Python, and remote modules (typically contributions by people who are not developing the core of ITK [1]) can also be wrapped and used [2]. ITK Python is a little less pythonic when it comes to using ITK filters but we are working on improving this.
So to answer your question a bit more specifically, I would say that SimpleITK is probably slightly easier to use for someone who is used to writing Python code and just want to use some ITK functions that are available in SimpleITK. ITK Python is great for people who want to access ITK functionalities that are not available in SimpleITK (registration I think), people who have used ITK in C++ but like to use Python because of the rapid prototyping and fast development that the language provide, or if one wants to contribute new functionalities through the development of a remote module
[1] But would be welcome to.
[2] So far, ITKTextureFeatures and ITKIsotropicWavelets have been wrapped.
[3] https://itk.org/ITKSoftwareGuide/html/Book1/ITKSoftwareGuide-Book1ch9.html#x48-1460009
Neat! I’ve been using SimpleITK for a couple of years and I used ITK with C++ once, so I guess I’m a good candidate to try it out. Any plans to include the itk module in Slicer?
If you compile Slicer on your own, there is an option to build ITK Python (Slicer_BUILD_ITKPython) in CMake that can be turned on. You can then access ITK Python from the Slicer Python console of in Python scripts. There is no current plans to create a module like the one of SimpleITK to directly embed all the ITK filters in the user interface of Slicer (I think SimpleITK does this well).
Hi Fernando,
To add to Francois’ remarks, a 3D Slicer extension is planned to install via the 3D Slicer Extension Manager or that other extensions can use as a dependency.
Regarding ITK and SimpleITK, SimpleITK has some similar methods, e.g. GetArrayFromImage and GetArrayViewFromImage. The relative differences are
itk:
– Covers the entire toolkit, including processing with PointSet’s, Mesh’s, SpecializedCoordinatesImage, etc.
– Retains the pipeline so very large images can be analyzed through streamed processing
– The wrapping and packaging for externally developed modules is straightforward and can be built on free GitHub CI services (documentation for this is in progress)
SimpleITK:
– It has a procedural interface, which is more intuitive for some folks who only have experience with Matlab.
Something is missing…
>>> median = itk.MedianImageFilter.New(image, Radius = 2)
Traceback (most recent call last):
File “”, line 1, in
File “/Library/Python/2.7/site-packages/itkTemplate.py”, line 416, in New
raise RuntimeError(“No suitable template parameter can be found.”)
RuntimeError: No suitable template parameter can be found.
Thanks for testing the project. Could you give more information? How did you install ITK? Would you be able to share the image you are using so I could reproduce your error?
I’m having a similar issue. What I did:
conda create -n itk3 # with Python 3
source activate itk3
conda install -c conda-forge itk # or pip install itk
python
And in python:
>>> import itk
>>> image = itk.imread(‘P1060807.JPG’)
>>> median = itk.MedianImageFilter.New(image, Radius = 2)
Traceback (most recent call last):
File “”, line 1, in
File “/Users/fernando/anaconda/lib/python3.6/site-packages/itkTemplate.py”, line 416, in New
raise RuntimeError(“No suitable template parameter can be found.”)
RuntimeError: No suitable template parameter can be found.
In a conda env with Python 2, I could install itk but not import it.
Regarding the conda-forge itk package, there was an older package that just provided the libraries for C++ development which has been renamed. The new package provides the Python bindings. This is being resolved. It is also possible to install the ITK Python package in conda via pip.
On a Mac I typed:
sudo easy_install pip
sudo pip install itk
I used the following png file:
wget https://upload.wikimedia.org/wikipedia/commons/7/70/Example.png
$ python
Python 2.7.10 (default, Oct 23 2015, 19:19:21)
[GCC 4.2.1 Compatible Apple LLVM 7.0.0 (clang-700.0.59.5)] on darwin
Type “help”, “copyright”, “credits” or “license” for more information.
>>> import itk
>>> image = itk.imread(“Example.png”)
>>> median = itk.MedianImageFilter.New(image, Radius = 2)
Traceback (most recent call last):
File “”, line 1, in
File “/Library/Python/2.7/site-packages/itkTemplate.py”, line 416, in New
raise RuntimeError(“No suitable template parameter can be found.”)
RuntimeError: No suitable template parameter can be found.
I forgot to say that I’m using macOS Sierra 10.12.6.
Dominque and Fernando: Thanks for reporting the problem you encountered. It is not due to a bug but to the fact that the median image filter cannot process RGB(A) images. In this case you need to convert your input image to a grayscale image first, or split the components before merging them back together. Converting the RGB(A) image to a grayscale image can be done with ITKRGBToLuminanceImageFilter. You can add this line of code in the example `grayscale=itk.RGBToLuminanceImageFilter.New(image)` before calling the median image filter and update the median image filter call to use the grayscale image.
Converting to grayscale worked, but I wish the error message was more descriptive. Thanks François
Indeed, the error message need to be improved to give clues to the user of how to solve the problem.
An issue has been created here to improve the error message:
https://issues.itk.org/jira/browse/ITK-3564
What’s a ‘vnl vector’?
@Aron: Great question. VNL vector are some of the data structures used by ITK to represent arrays and matrices. The name come from the library providing support for it. See http://vxl.sourceforge.net/
Hi. I might be a little late in this conversation, but what if I am interested in going from ITK image to numpy array? Is there an easy way to do that? Thanks,
Hi Matheus,
Yes to go from ITK image to NumPy array, use
array = itk.GetArrayFromImage(image)
or, with ITK 5,
array = itk.array_from_image(image)
See the recent release announcement for more details:
https://blog.kitware.com/itk-5-0-beta-1-pythonic-interface/