Reading DICOM images, and non-image SOP classes in JavaScript and Python
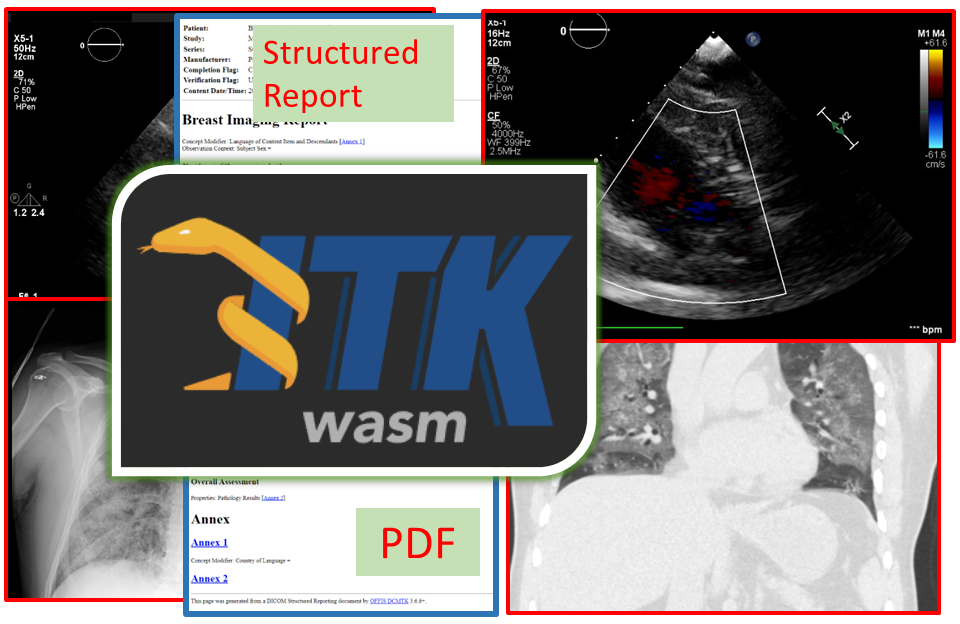
Introduction
itk-wasm uses web-assembly to bring ITK’s high performance imaging algorithms to platforms such as the browser, Python, and Node.js through a single source package and uniform API.
A sub-package of this library, itkwasm-dicom, was recently released which provides essential support for reading of medical data using the DICOM standard for storing images and other diagnostic information. This work was primarily motivated due to the need for bringing DICOM file reading capabilities to server-side and client-side JavaScript and Python applications. The JavaScript name for this package is @itk-wasm/dicom and Python name is itkwasm-dicom.
Related Tools
Other existing open-source software that support DICOM IO include C++ packages such as DCMTK and GDCM. GDCM also has additional wrappers to support languages (C#, Java, Python), but it doesn’t support some important non-image related classes such as presentation states and structured reports. Python based packages such as pydicom are built upon GDCM and hence inherit similar limitations. When it comes to JavaScript, there have been some efforts to cross compile DCMTK to JavaScript (commontk/dcmjs) which is currently not maintained. Similarly, dcmjs-org/dcm-js is a pure JavaScript library being written for reading DICOMs but is currently in its early stages.
In our limited survey, we found that DCMTK has one of the most comprehensive support for DICOM even though some classes such as color softcopy presentation state (CSPS) are not supported out of the box. We, therefore, build our DICOM capabilities in itk-wasm through web assembly compilation of DCMTK using WASI and Emscripten.
We have recently added support for non-imaging related DICOM SOP (Service-Object Pair) classes such as structured reports, encapsulated PDFs, and color / grayscale presentation states. In the following sections, we list the supported SOP classes and how you can start using itk-wasm and itk-wasm-dicom with your JavaScript and Python applications.
Supported SOP Classes
The DICOM standard defines how various medical media, such as images, reports, waveforms, annotations, and measurements, are to be stored and accessed (SOP classes).
Since itk-wasm builds upon ITK, it already has significant support for reading DICOM and other medical image file formats. ITK’s DICOM reading capabilities are mainly supported through third-party libraries, namely GDCM and DCMTK. While it is a considerable task to comprehensively support the entire gamut of classes, we have tested our support for the following classes:
List of supported DICOM SOP image classes:
- Computed Tomography (CT)
- Enhanced CT Image
- Magnetic Resonance (MR)
- Enhanced MR Image
- Computed Radiography Image (CR)
- Digital X-Ray Image (DX)
- Digital Mammography X-Ray Image (MG)
- Positron Emission Tomography Image (PET)
- Nuclear Medicine Image (NM)
- Ultrasound Image (US)
- Ultrasound Multi-frame Image
- Secondary Capture Image (SC)
- Multi-frame Secondary Capture Image
- Segmentation Storage (SEG)
The DICOM image storage formats are supported through ITK’s IO modules (subsequently through linked DCMTK and GDCM libraries) compiled into web-assembly for itk-wasm.
List of supported DICOM SOP non-image classes:
- Basic, Enhanced, Comprehensive Structured Report (SR)
- X-Ray Radiation Dose Report (SR)
- Key Object Selection Document (SR)
- Encapsulated PDF Storage
- Grayscale Softcopy Presentation State Storage SOP Class (GSPS)
- Color Softcopy Presentation State Storage SOP Class (CSPS)
An updated list of supported classes is maintained here: DICOM (itk.org)
Limitations
Support for reading color softcopy presentation states (CSPS) is currently preliminary. Particularly, the color profile (ICC) is extracted from the presentation state file and provided through the output JSON as a property. It is left to the calling application to decide how to apply the color transformations to the referenced image.
The physical transformation of the image is performed based on transformations (flip, rotation) indicated in the presentation state tags and provided as additional output along with the JSON. It is surprisingly difficult to find publicly available real-world examples of CSPS, which currently limits our capability to test and expand the support for the CSPS format. If you encounter issues/bugs during your usage, we will be happy to know what didn’t work for you. Developers are welcome to report the same on the GitHub repository issue tracker.
Structured reports and other document formats such as key object selection and x-ray dose reports are rendered as HTML using DCMTK’s capabilities. This is a basic way of rendering the reports. Whereas, there could be better ways to present them. Further investigation is required for use of report templates.
Getting Started
Using with JavaScript
// installing dependencies
$ npm install itk-wasm
$ npm install @itk-wasm/dicom
$ npm install fs
// using itkwasm_dicom
import fs from 'fs'
import {structuredReportToTextNode} from '@itk-wasm/dicom'
const fileName = 'comprehensive-SR.dcm'
const srFilePath = '/path-to/my-data/' + fileName
const dicomFileBuffer = fs.readFileSync(testFilePath)
const srArrayBuffer = new Uint8Array(dicomFileBuffer)
const { srOutputText } = await structuredReportToTextNode({ data: srArrayBuffer, path: fileName })
console.log('Output of structured report as text: ', srOutputText)
Using with Python
pip install itkwasm itkwasm-dicom --upgrade
import itkwasm
import itkwasm_dicom
output = itkwasm_dicom.structured_report_to_html(
"./path_to/structured_report.dcm", css_file = "./path_to/test-style.css")
print(output)
with open('SR-to-html-output.html', 'w') as f:
f.write(output)
Future Work
More DICOM support to meet your needs
We plan to continue testing and expanding the DICOM support through itk-wasm in the near future. Stay tuned for future updates and posts on how to use itk-wasm with medical data and DICOM image/non-image data formats. itkwasm-dicom is currently hosted within the itk-wasm repository. In the future, we plan to move the package to its own repository (ITK-DICOM) as a dedicated external ITK-module that can be built with ITK C++ as well as web assembly.
If you have any questions, issues, or a feature request in itk-wasm or itkwasm-dicom, feel free to post an issue on the GitHub page. We would love to hear your feedback.