Vcpkg: A tool to build open source libraries on Windows
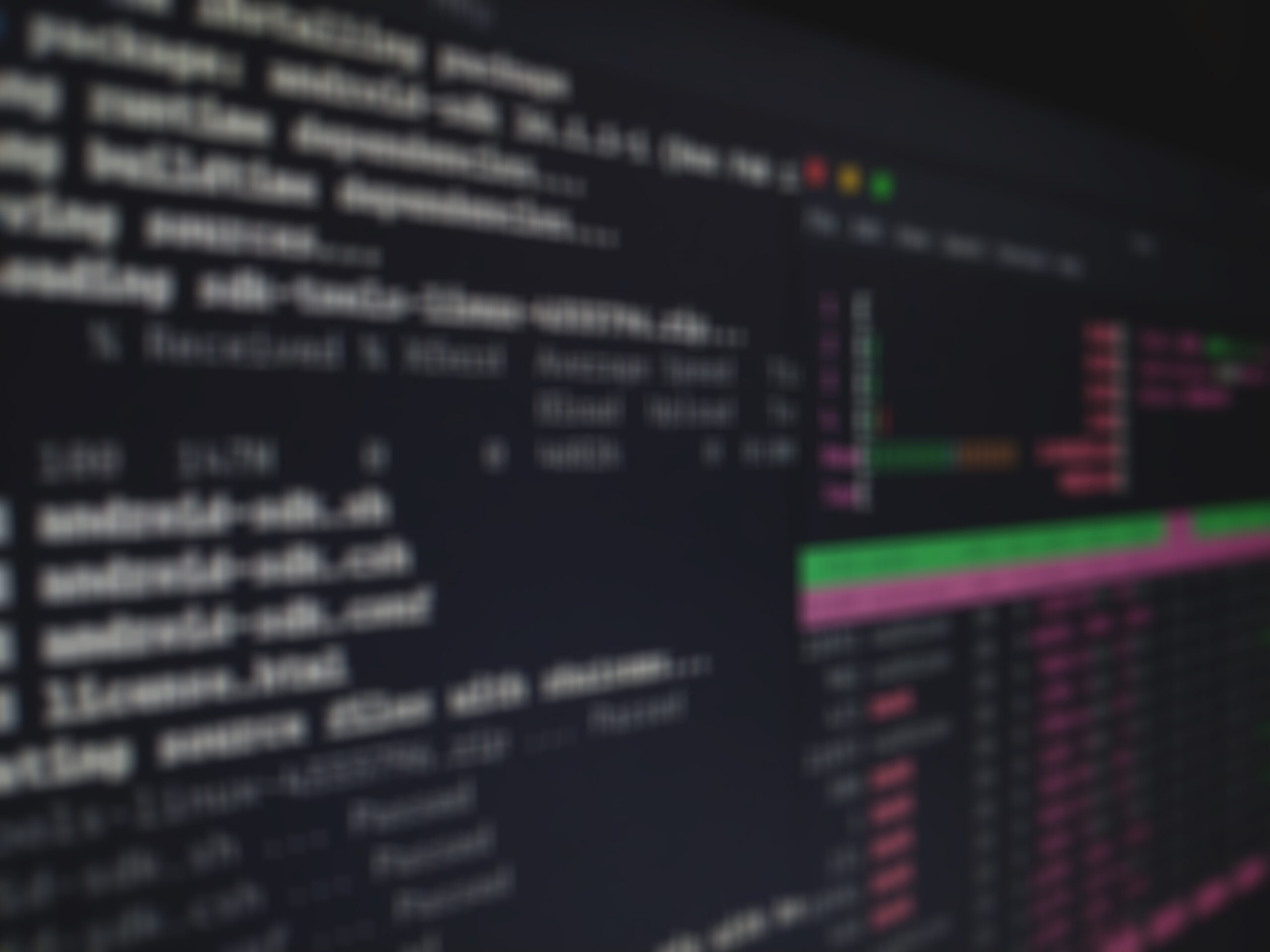
In this post we describe Vcpkg, a tool created by Microsoft that helps acquire and build open source C and C++ libraries.
On Windows, downloading, building, and installing the libraries that a project requires can be difficult and time-consuming. First, you must locate and download each library from its own server or repository. Next, you must configure and build the libraries, each of which may use its own build system. Finally, you must set up your project to be able to find each library’s headers and to link to each library.
Vcpkg attempts to simplify the process of installing libraries on Windows to a single command line. Furthermore, by setting up the build environment using a CMake toolchain file, Vcpkg eliminates the need to manually define paths to libraries when configuring CMake-based projects.
We experimented using Vcpkg while developing a commercial project. The project relies on gRPC, an open source RPC framework, which proved difficult to build manually on Windows.
At the time of this writing, over 600 libraries are available in the Vcpkg ecosystem, including popular ones such as Boost, OpenCV, OpenSSL, and zlib. Vcpkg requires Visual Studio 2015 Update 3 or Visual Studio 2017.
Example: Install and use zlib
Vcpkg is best demonstrated by an example. Follow the steps below to install zlib and build a project that uses the installed zlib.
Install Visual Studio
Download and install Visual Studio from https://www.visualstudio.com/.
Set up Vcpkg:
- Clone the repository at https://github.com/Microsoft/vcpkg.git
- Run:
C:\dev\vcpkg> .\bootstrap-vcpkg.bat
The bootstrap script builds vcpkg.exe and vcpkgmetricsuploader.exe and copies them into C:\dev\vcpkg and C:\dev\vcpkg\scripts, respectively. vcpkgmetricsuploader.exe sends usage information about vcpkg.exe to Microsoft.
Install 64-bit zlib:
C:\dev\vcpkg> vcpkg search zlib liblzma 5.2.3-2 Compression library with an API similar to that of zlib. zlib 1.2.11-3 A compression library If your library is not listed, please open an issue at and/or consider making a pull request: https://github.com/Microsoft/vcpkg/issue
C:\dev\vcpkg>vcpkg install zlib --triplet x64-windows The following packages will be built and installed: zlib[core]:x64-windows Starting package 1/1: zlib:x64-windows Building package zlib:x64-windows... -- CURRENT_INSTALLED_DIR=C:/dev/vcpkg/installed/x64-windows -- DOWNLOADS=C:/dev/vcpkg/downloads -- CURRENT_PACKAGES_DIR=C:/dev/vcpkg/packages/zlib_x64-windows -- CURRENT_BUILDTREES_DIR=C:/dev/vcpkg/buildtrees/zlib -- CURRENT_PORT_DIR=C:/dev/vcpkg/ports/zlib/. -- Using cached C:/dev/vcpkg/downloads/zlib1211.tar.gz -- Testing integrity of cached file... -- Testing integrity of cached file... OK -- Extracting done -- Applying patch C:/dev/vcpkg/ports/zlib/cmake_dont_build_more_than_needed.patch -- Applying patch C:/dev/vcpkg/ports/zlib/cmake_dont_build_more_than_needed.patch done -- Downloading https://github.com/ninja-build/ninja/releases/download/v1.8.2/ninja-win.zip... -- Downloading https://github.com/ninja-build/ninja/releases/download/v1.8.2/ninja-win.zip... OK -- Testing integrity of downloaded file... -- Testing integrity of downloaded file... OK -- Configuring x64-windows-rel -- Configuring x64-windows-rel done -- Configuring x64-windows-dbg -- Configuring x64-windows-dbg done -- Build x64-windows-rel -- Build x64-windows-rel done -- Build x64-windows-dbg -- Build x64-windows-dbg done -- Installing: C:/dev/vcpkg/packages/zlib_x64-windows/share/zlib/copyright -- Performing post-build validation -- Performing post-build validation done Building package zlib:x64-windows... done Installing package zlib:x64-windows... Installing package zlib:x64-windows... done Elapsed time for package zlib:x64-windows: 29.85 s
Total elapsed time: 29.85 s
The package zlib is compatible with built-in CMake targets:
find_package(ZLIB REQUIRED) target_link_libraries(main PRIVATE ZLIB::ZLIB)
Build the project:
CMakeLists.txt:
cmake_minimum_required(VERSION 3.0) project(example) find_package(ZLIB REQUIRED) add_executable(example example.cpp) target_link_libraries(example ZLIB::ZLIB)
example.cpp:
#include <iostream> #include <zlib.h> int main(int argc, char *argv[]) { std::cout << zlibVersion() << std::endl; return 0; }
Configure and build:
Run CMake, specifying the Vcpkg toolchain file:
C:\dev\example-build> cmake.exe -DCMAKE_TOOLCHAIN_FILE=C:/dev/vcpkg/scripts/buildsystems/vcpkg.cmake -G "Visual Studio 15 2017 Win64" ..\example
For reference, the configuration can be seen in the CMake GUI:
C:\dev\example-build> cmake-gui.exe .
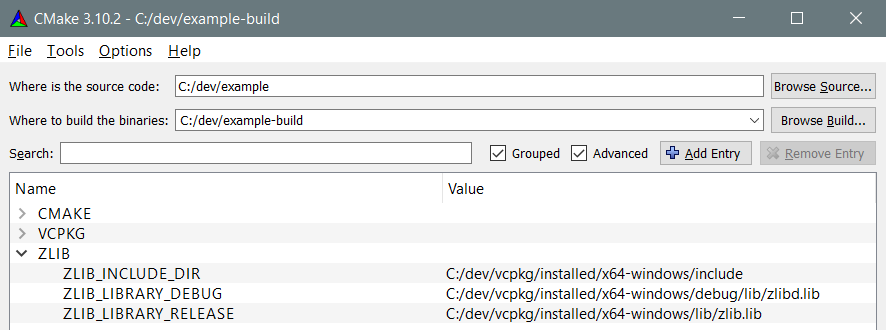
Build the project:
C:\dev\example-build> cmake.exe --build .
Note that the required DLLs, zlibd1.dll in this example, are automatically copied to the output directory.
Run the program:
C:\dev\example-build> Debug\example.exe 1.2.11
How it works
- Each library in the Vcpkg ecosystem contains a portfile—a CMake script—that is responsible for fetching sources or binaries, configuring builds, and installing headers and libraries into the correct locations. See zlib/portfile.cmake for an example.
- Because portfiles are CMake scripts, there’s no inherent restriction on which build system libraries can use.
- Vcpkg’s CMake toolchain file and Visual Studio integration configure the environment so that the installed headers and libraries are available when building projects.
- Based on the specified CMake generator, the vcpkg.cmake toolchain file determines the target architecture and platform and updates CMAKE_PREFIX_PATH and CMAKE_LIBRARY_PATH so that the find_package and find_library CMake commands locate the appropriate config files and libraries. Additionally, it overrides add_executable to add a POST_BUILD step that copies dependent shared libraries to the output directory alongside the executable.
Building static libraries
By default, Vcpkg builds dynamic libraries. To build static libraries, specify the –triplet x64-windows-static option when running vcpkg install. Alternatively, you can set this value in the VCPKG_DEFAULT_TRIPLET environment variable.
When configuring a project to use static libraries, it’s currently necessary to explicitly specify the static triplet, like: -DVCPKG_TARGET_TRIPLET:STRING=x64-windows-static. Additionally, your project should be configured to use the static C runtime library.
Kitware packages
The Visualization Toolkit (VTK) and the Insight Toolkit (ITK) are already available through Vcpkg.
Conclusion
In this post we introduced Vcpkg and showed how to install libraries using Vcpkg. Additionally, we provided an example of a CMake-based project that uses a library installed through Vcpkg.
Vcpkg provides a convenient way to install and use open source C and C++ libraries on Windows. If you’re building a project that depends on more than a few libraries, using Vcpkg might save you some time.
Hi! Thank you for such a great article. One small detail could be fixed though: “U+2013 : EN DASH”(–) is used instead of double “U+002D : HYPHEN-MINUS”(–), which prevents code from executed properly (when copy-pasted).
We will fix it, thanks!
Thanks Anton, we converted the examples and references to variables and command line arguments to be in fixed font. Let us know if that works better.
It also look like there is an issue with the rendering of “greater than” and “smaller than” in the examples, this will fix fixed shortly.
Formatting issues have been addressed.